Getters
The Biomes client will periodically fetch these getter functions to display information about your experience to a player who has opted-in to your experience by registering your hooks.
Experience Details
//Name of Experience
function getDisplayName() external view returns (string memory);
//Optional: Dynamic Instructions to Show Player
function getStatus() external view returns (string memory);
//Optional: Timestamp When Game Starts or Ends
function getCountdownEndTimestamp() external view returns (uint256);
//Optional: Blocknumber Before Game Starts or Ends
function getCountdownEndBlock() external view returns (uint256);
//Optional: What Happens When Player Unregisters Hooks/Delegations
function getUnregisterMessage() external view returns (string memory);
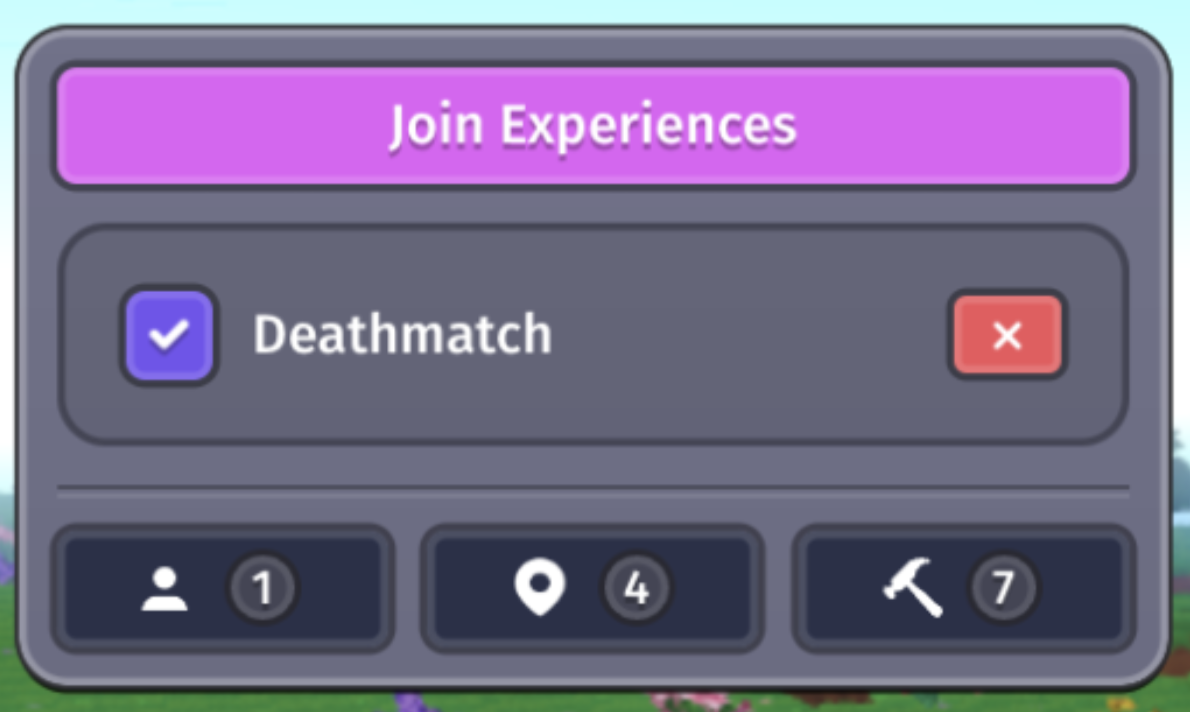
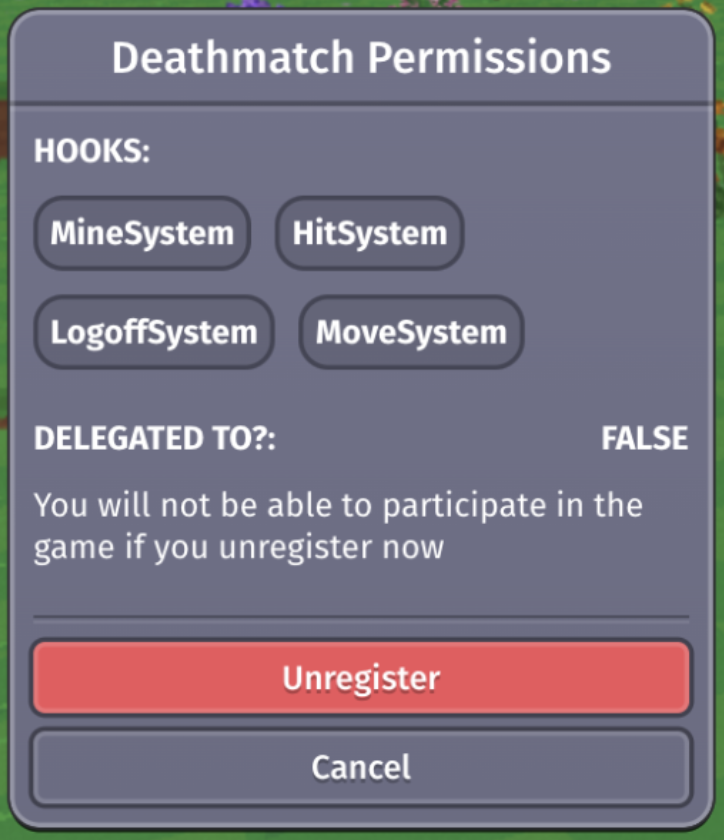
Avatars
//Avatars - Purple Beams Will Be Displayed On Them
function getAvatars() external view returns (bytes32[] memory);
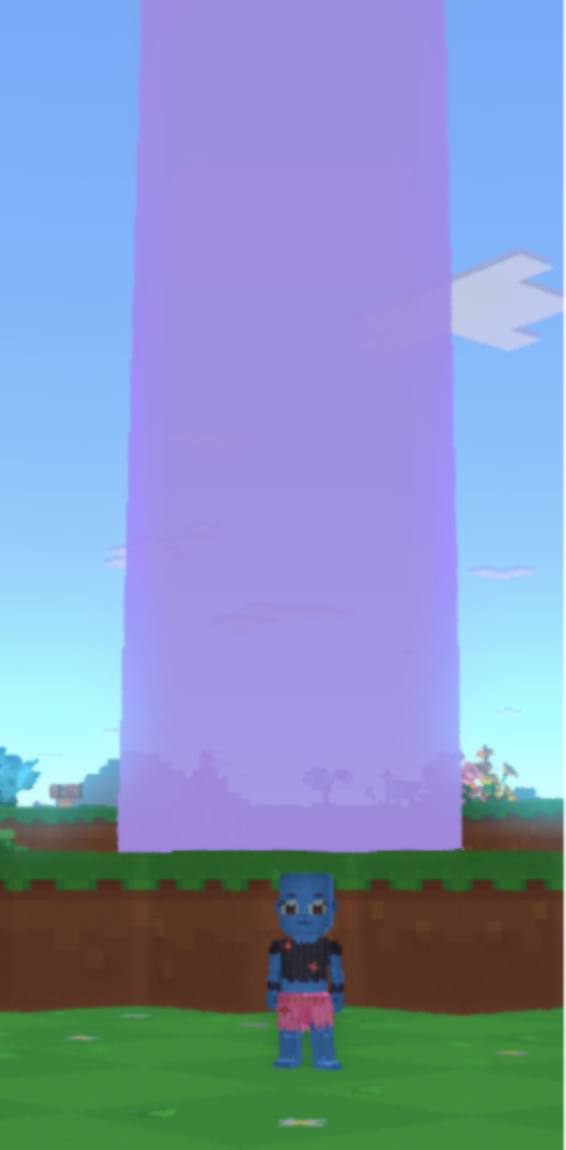
Areas
struct Area {
VoxelCoord lowerSouthwestCorner;
VoxelCoord size;
}
struct NamedArea {
string name;
Area area;
}
//Areas - Red Borders Will Be Displayed Around Them
function getAreas() external view returns (NamedArea[] memory);
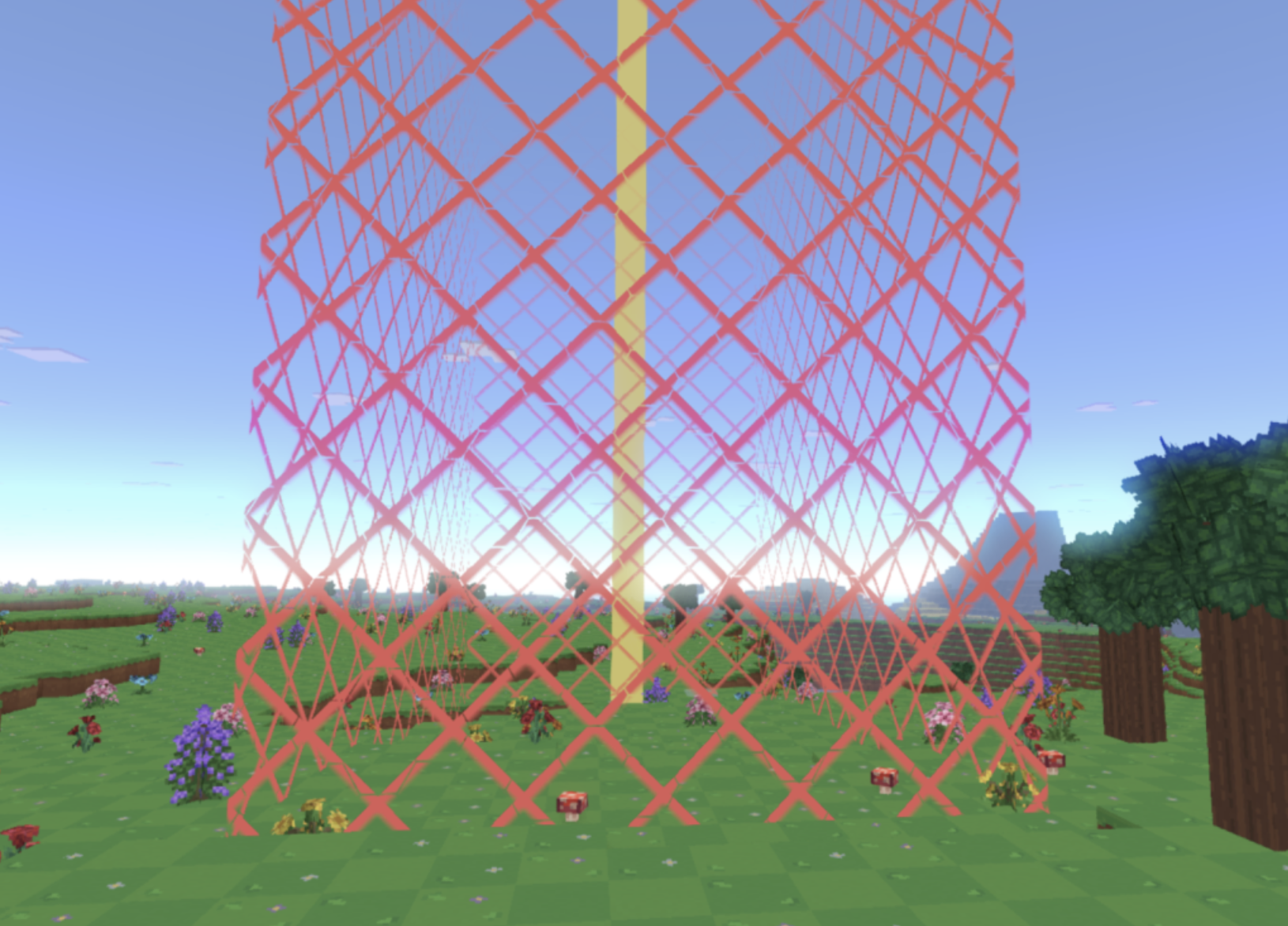
Builds
struct Build {
uint8[] objectTypeIds;
VoxelCoord[] relativePositions;
}
struct BuildWithPos {
uint8[] objectTypeIds;
VoxelCoord[] relativePositions;
VoxelCoord baseWorldCoord;
}
struct NamedBuild {
string name;
Build build;
}
struct NamedBuildWithPos {
string name;
BuildWithPos build;
}
//Builds - Players Will Be Able To Place Their Outlines
function getBuilds() external view returns (NamedBuild[] memory);
//Builds Which Must Be Placed At A Specific Position
function getBuildsWithPos() external view returns (NamedBuildWithPos[] memory);
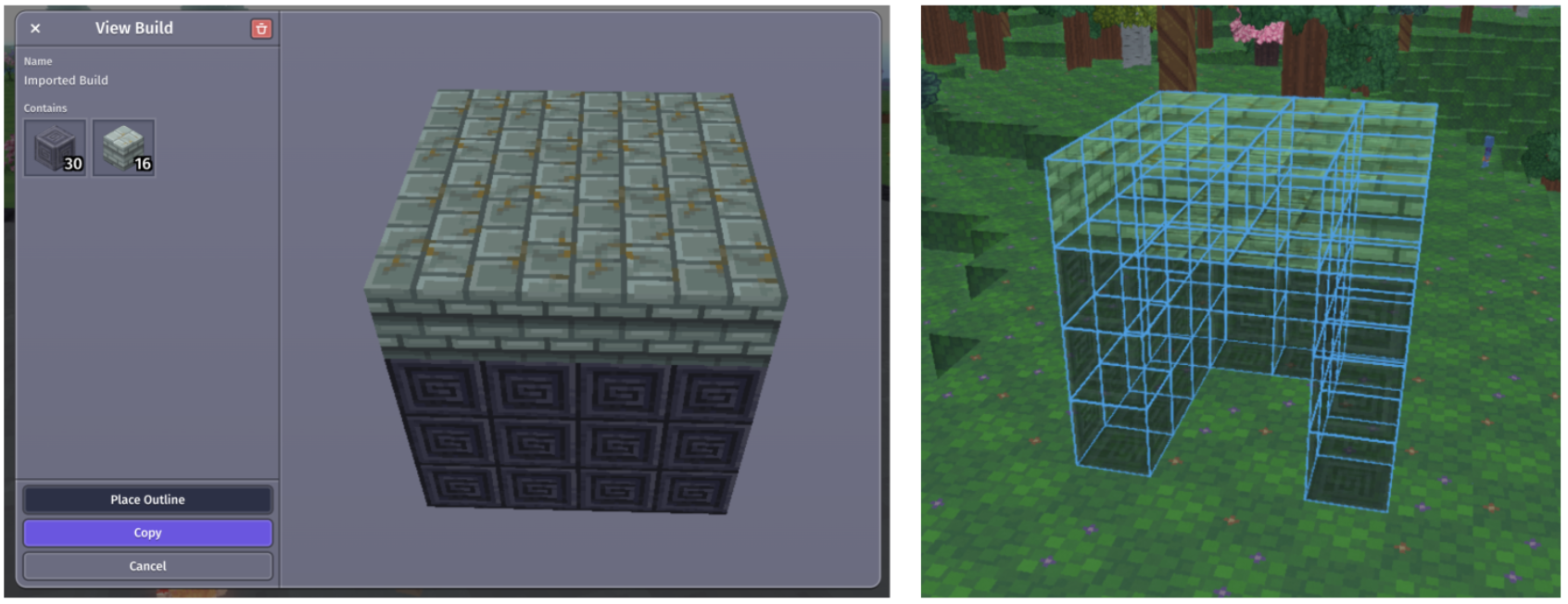